- 강제이동
- ViewBag
- 말줄임표시
- php 캐쉬제거
- XSS PHP
- asp.net dropdownlist
- XSS방어
- javascript 바코드 생성
- Mac Oracle
- javascript redirection
- 파일업로드 유효성체크
- 404에러페이지
- SSD 복사
- 하드 마이그레이션
- django 엑셀불러오기
- javascript 바코드스캔
- 바코드 스캔하기
- 파일업로드 체크
- javascript 유효성체크
- ViewData
- jquery 바코드생성
- 맥 오라클설치
- asp.net Select
- asp.net core Select
- 하드 윈도우 복사
- ASP.Net Core 404
- jquery 바코드
- 타임피커
- TempData
- 바코드 생성하기
웹개발자의 기지개
[ASP.Net Core] SignalR 을 이용한 실시간 채팅 본문
[ASP.Net Core] SignalR 을 이용한 실시간 채팅
http://portfolio.wonpaper.net 2023. 12. 24. 23:49
ASP.NET Core SignalR 시작하기
이 자습서에서는 ASP.NET Core SignalR을 사용하는 채팅 앱을 만듭니다.
learn.microsoft.com
ASP.Net Core SignalR 공식 자습서 소스에는 Page Razor 방식으로 예제를 만들어 놓았는데,
필자는 MVC 방식으로 변경해서 돌려 보았다.
우선 Github 에 필자의 소스를 모두 올려 올려놓았다.
https://github.com/wonpaper/ASPNetCore_SignalR_SimpleChat
GitHub - wonpaper/ASPNetCore_SignalR_SimpleChat
Contribute to wonpaper/ASPNetCore_SignalR_SimpleChat development by creating an account on GitHub.
github.com
필자는 ASP.Net Core6 을 기준으로 구성하였다.
SimpleChat 이라는 프로젝트를 하나 만들고,
[ Program.cs ]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
|
using SimpleChat.Models.Chat;
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddSignalR();
builder.Services.AddControllersWithViews();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.MapHub<ChatHub>("/chatHub");
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
app.Run();
|
cs |
SimpleChat 이라는 프로젝트를 하나 만들고,
builder.Services.AddSignalR();
app.MapHub<ChatHub>("/chatHub");
추가한다.
[ /Models/Chat/ChatHub.cs ]
1
2
3
4
5
6
7
8
9
10
11
12
|
using Microsoft.AspNetCore.SignalR;
namespace SimpleChat.Models.Chat
{
public class ChatHub : Hub
{
public async Task SendMessage(string user, string message)
{
await Clients.All.SendAsync("ReceiveMessage",user, message);
}
}
}
|
cs |
그다음에는
MS로부터 클라이언트 js 파일들을 불러온다.
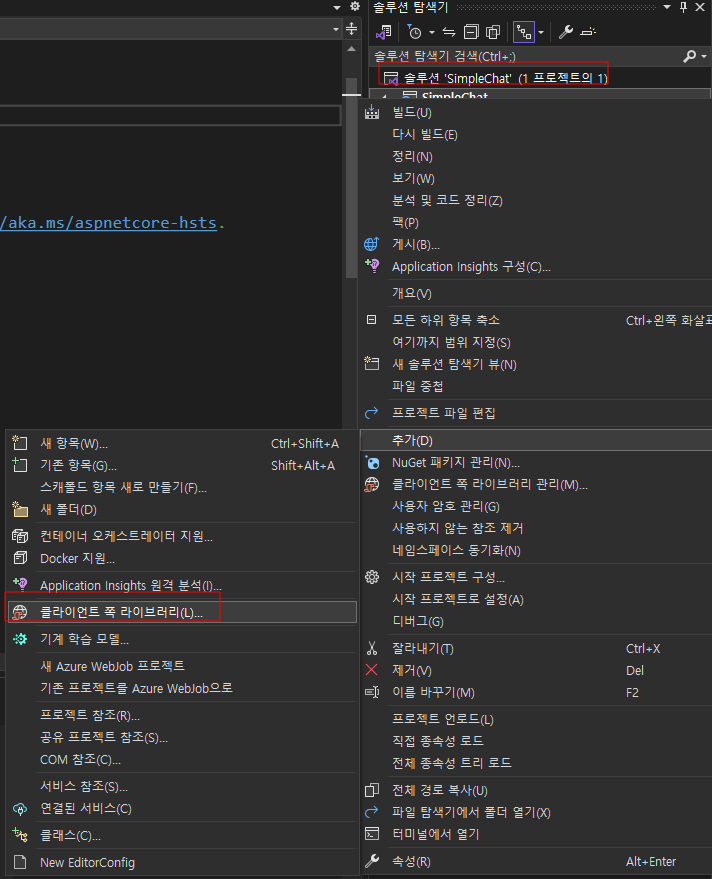
[ /wwwroot/js/chat.js ]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
|
var connection = new signalR.HubConnectionBuilder().withUrl("/chatHub").build();
//Disable the send button until connection is established.
document.getElementById("sendButton").disabled = true;
connection.on("ReceiveMessage", function (user, message) {
var li = document.createElement("li");
document.getElementById("messagesList").appendChild(li);
// We can assign user-supplied strings to an element's textContent because it
// is not interpreted as markup. If you're assigning in any other way, you
// should be aware of possible script injection concerns.
li.textContent = `${user} says ${message}`;
});
connection.start().then(function () {
document.getElementById("sendButton").disabled = false;
}).catch(function (err) {
return console.error(err.toString());
});
document.getElementById("sendButton").addEventListener("click", function (event) {
var user = document.getElementById("userInput").value;
var message = document.getElementById("messageInput").value;
connection.invoke("SendMessage", user, message).catch(function (err) {
return console.error(err.toString());
});
event.preventDefault();
});
|
cs |
그 다음으로는 MVC 기본형인 View html 파일 구성이다.
[ /Views/Home/Index.cshtml ]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
|
@{
ViewData["Title"] = "Home Page";
}
<div class="container">
<div class="row"> </div>
<div class="row">
<div class="col-2">User</div>
<div class="col-4"><input type="text" id="userInput" /></div>
</div>
<div class="row">
<div class="col-2">Message</div>
<div class="col-4"><input type="text" id="messageInput" /></div>
</div>
<div class="row"> </div>
<div class="row">
<div class="col-6">
<input type="button" id="sendButton" value="Send Message" />
</div>
</div>
</div>
<div class="row">
<div class="col-12">
<hr />
</div>
</div>
<div class="row">
<div class="col-6">
<ul id="messagesList"></ul>
</div>
</div>
@section scripts {
<script src="~/js/signalr/dist/browser/signalr.js"></script>
<script src="~/js/chat.js"></script>
}
|
cs |
'ASP.NET > ASP.NET Core' 카테고리의 다른 글
[ASP.Net Core] 대용량 파일 업로드 시키기 (1) | 2024.03.08 |
---|---|
[ASP.Net Core] CSS 파일 수정시 실시간으로 반영시키기 (0) | 2024.03.06 |
[ASP.Net Core] Entity Framework Core 2 - LINQ ( Include , ThenInclude ) (0) | 2023.09.30 |
[ASP.Net Core] SeriLog 로그 작업하기 (0) | 2023.09.13 |
[ASP.Net Core] Radio 버튼 처리해보기 (0) | 2023.07.22 |