- asp.net core Select
- 404에러페이지
- asp.net core swagger
- ASP.Net Core 404
- jquery 바코드생성
- 맥 오라클설치
- SSD 복사
- ViewData
- 바코드 스캔하기
- 하드 마이그레이션
- asp ftp
- php 캐쉬제거
- 말줄임표시
- 바코드 생성하기
- django 엑셀불러오기
- javascript 바코드 생성
- swagger 500 error
- asp.net Select
- TempData
- Mac Oracle
- 하드 윈도우 복사
- javascript redirection
- ViewBag
- asp.net dropdownlist
- 강제이동
- XSS방어
- XSS PHP
- 타임피커
- 원격ftp
- simpe ftp
웹개발자의 기지개
[ASP.Net Core MVC] Entity Framework Core 1 - 다대다관계 본문
[ASP.Net Core MVC] Entity Framework Core 1 - 다대다관계
http://portfolio.wonpaper.net 2022. 6. 4. 06:49ORM 인 Entiry Framework Core 를 바탕으로 다대다관계를 구성해보도록 하자.
다대다 관계는 보통의 경우 1:다:1 형태로 임의의 중간 테이블을 별도로 만들고 이를 연결하는 형태로 구성한다.
이때 1:다는 각각 ForeignKey 형태로 연결시킨다.
관련 예제는 MS 의 ASP.Net Core 자습서를 참고 하였다.
https://docs.microsoft.com/ko-kr/aspnet/core/data/ef-mvc/intro?view=aspnetcore-3.1
자습서: ASP.NET MVC 웹앱에서 EF Core 시작
이 페이지는 Contoso University 샘플 EF/MVC 앱을 빌드하는 방법을 설명하는 자습서 시리즈 중 첫 번째입니다.
docs.microsoft.com
깃허브 전체 소스는 아래의 주소와 같다.
https://github.com/dotnet/AspNetCore.Docs/tree/main/aspnetcore/data/ef-mvc/intro/samples/cu-final
GitHub - dotnet/AspNetCore.Docs: Documentation for ASP.NET Core
Documentation for ASP.NET Core. Contribute to dotnet/AspNetCore.Docs development by creating an account on GitHub.
github.com
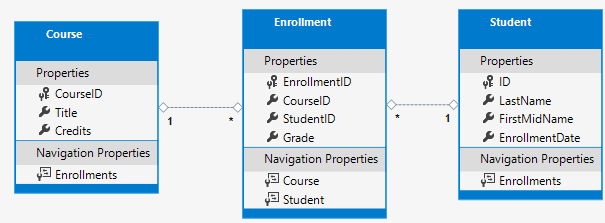
상기 이미지와 같애 Course 와 Student 간의 다대다 관계를 Enrollment 엔터티로 연결하여 1:다:1 처리를 하고 있다.
[ /Models/Course.cs ]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations.Schema;
namespace ContosoUniversity.Models
{
public class Course
{
[DatabaseGenerated(DatabaseGeneratedOption.None)]
public int CourseID { get; set; }
public string Title { get; set; }
public int Credits { get; set; }
public ICollection<Enrollment> Enrollments { get; set; }
}
}
|
cs |
[ /Models/Student.cs ]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
using System;
using System.Collections.Generic;
namespace ContosoUniversity.Models
{
public class Student
{
public int ID { get; set; }
public string LastName { get; set; }
public string FirstMidName { get; set; }
public DateTime EnrollmentDate { get; set; }
public ICollection<Enrollment> Enrollments { get; set; }
}
}
|
cs |
[ /Models/Enrollment.cs ]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
namespace ContosoUniversity.Models
{
public enum Grade
{
A=1, B, C, D, F
}
public class Enrollment
{
public int EnrollmentID { get; set; }
public int CourseID { get; set; }
public int StudentID { get; set; }
public Grade? Grade { get; set; }
public Course Course { get; set; }
public Student Student { get; set; }
}
}
|
cs |
[ /Data/SchoolContext.cs ]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
using ContosoUniversity.Models;
using Microsoft.EntityFrameworkCore;
namespace ContosoUniversity.Data
{
public class SchoolContext : DbContext
{
public SchoolContext(DbContextOptions<SchoolContext> options) : base(options)
{
}
public DbSet<Course> Courses { get; set; }
public DbSet<Student> Students { get; set; }
public DbSet<Enrollment> Enrollments { get; set; }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<Course>().ToTable("Course");
modelBuilder.Entity<Student>().ToTable("Student");
modelBuilder.Entity<Enrollment>().ToTable("Enrollment");
}
}
}
|
cs |
SchoolContext 는 DbContext 를 상속하고, 아래의 Startup.cs 내에 서비스항목에 추가 시켜준다.
아래의 Startup.cs 에서 31라인지점이다.
[ Startup.cs ]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
|
using ContosoUniversity.Data;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.HttpsPolicy;
using Microsoft.EntityFrameworkCore;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace ContosoUniversity
{
public class Startup
{
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
public IConfiguration Configuration { get; }
public void ConfigureServices(IServiceCollection services)
{
// 세션추가
services.AddSession();
services.AddControllersWithViews();
// DB Context추가
services.AddDbContext<SchoolContext>(options => options.UseSqlServer(Configuration.GetConnectionString("AppDb")));
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Home/Error");
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
// 세션사용
app.UseSession();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
});
}
}
}
|
cs |
[ appsettings.json ]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information"
}
},
"AllowedHosts": "*",
"ConnectionStrings": {
"AppDb": "Server=DESKTOP-ASIDMUS;Database=ContosoUniversity;Trusted_Connection=True;MultipleActiveResultSets=true"
}
}
|
cs |
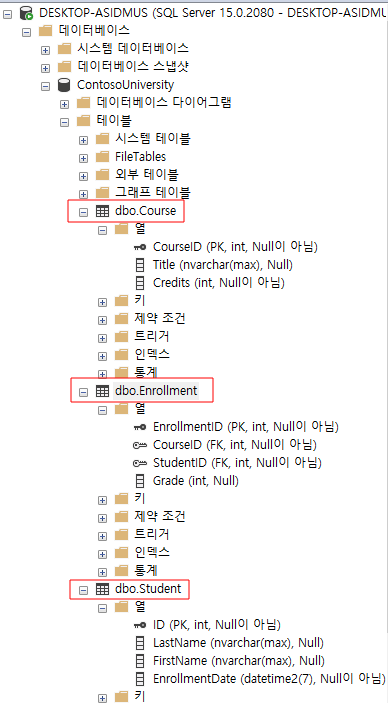
3개의 테이블은 아래의 소스로 스크립트파일로 정리해 놓았다.
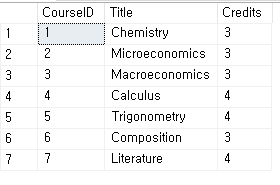
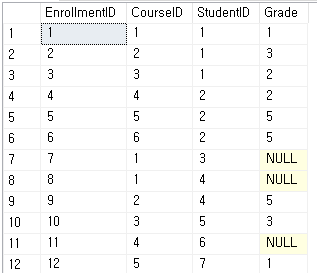
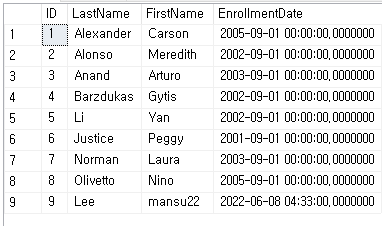
참고1 : https://docs.microsoft.com/ko-kr/aspnet/core/data/ef-mvc/read-related-data?view=aspnetcore-3.1
참고2 : https://dleunji.tistory.com/45?category=999005
참고3 : https://www.learnentityframeworkcore.com/relationships
참고4 : https://forum.dotnetdev.kr/t/ef-core-6-2/3716
참고5 : https://www.learnentityframeworkcore.com/configuration/many-to-many-relationship-configuration
'ASP.NET > ASP.NET Core' 카테고리의 다른 글
[ASP.Net Core MVC] Area 형태로 묶어서 사용하기 (0) | 2022.09.16 |
---|---|
[ASP.Net Core MVC] 앱프로젝트 재시작없이 페이지 변경 자동 새로고침 Razor.RuntimeCompilation (0) | 2022.09.16 |
[asp.net core MVC] 간단한 회원가입,로그인,로그아웃 (with DB, EntityFrameworkCore) (3) | 2020.05.16 |
[asp.net core MVC] ASP.NET Core 의 AddScoped AddTransient AddSingleton 수명 주기 (0) | 2020.03.27 |
[asp.net core MVC] AspNetCore Identity 상의 비밀번호 정책 설정 임의 변경하기 (0) | 2020.03.25 |