- ASP.Net Core 404
- ViewBag
- 404에러페이지
- 바코드 스캔하기
- asp ftp
- XSS PHP
- asp.net core Select
- Mac Oracle
- XSS방어
- 맥 오라클설치
- swagger 500 error
- django 엑셀불러오기
- 하드 윈도우 복사
- 하드 마이그레이션
- asp.net core swagger
- asp.net dropdownlist
- TempData
- 타임피커
- ViewData
- simpe ftp
- javascript 바코드 생성
- javascript redirection
- 강제이동
- asp.net Select
- 말줄임표시
- 원격ftp
- SSD 복사
- 바코드 생성하기
- jquery 바코드생성
- php 캐쉬제거
웹개발자의 기지개
[ASP.Net Core] Select박스 구현하기 - DropDownList 대분류,중분류 카테고리 [上] 본문
[ASP.Net Core] Select박스 구현하기 - DropDownList 대분류,중분류 카테고리 [上]
http://portfolio.wonpaper.net 2023. 2. 9. 01:41HTML 상의 Select박스를 구현해보자.
Create 상에서 Select 박스와 Edit 처리시에 선택되어진 Select 박스 두부분으로 구분하여 구현해보자.
Categories 대분류 테이블 , SubCategories 중분류 테이블로 구분
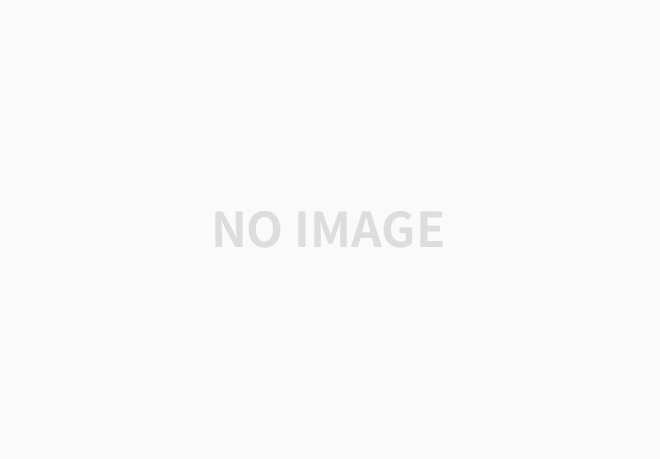
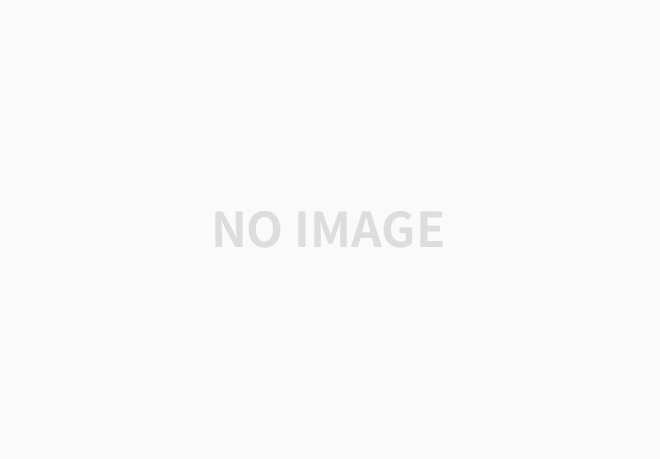
Entity Framwork 를 이용하는데, 먼저 DbContext 부터 시작하였다.
[ ApplicationDbContext.cs ]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
using FastFood.Models;
using Microsoft.AspNetCore.Identity;
using Microsoft.AspNetCore.Identity.EntityFrameworkCore;
using Microsoft.EntityFrameworkCore;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace FastFood.Repository
{
public class ApplicationDbContext : IdentityDbContext
{
public ApplicationDbContext(DbContextOptions<ApplicationDbContext> options): base(options)
{
}
public DbSet<Category> Categories { get; set; }
public DbSet<SubCategory> SubCategories { get; set; }
}
}
|
cs |
위 소스에서 IdentityDbContext 가 아니고, DbContext 를 상속하여 처리해도 된다.
[ Category, SubCategory 클래스 ]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
|
namespace FastFood.Models
{
public class Category
{
[Key]
public int Id { get; set; }
[Required]
public string Title { get; set; }
[JsonIgnore]
[IgnoreDataMember]
public ICollection<Item> Items { get; set; }
[JsonIgnore]
[IgnoreDataMember]
public ICollection<SubCategory> SubCategories { get; set; }
}
public class SubCategory
{
[Key]
public int Id { get; set; }
[Required]
public string Title { get; set; }
public int CategoryId { get; set; } // FK
public Category Category { get; set; }
public ICollection<Item> Items { get; set; }
}
public class Item
{
[Key]
public int Id { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public string Image { get; set; }
public double Price { get; set; }
public int CategoryId { get; set; }
public Category Category { get; set; }
public int SubCategoryId { get; set; }
public SubCategory SubCategory { get; set; }
}
}
|
cs |
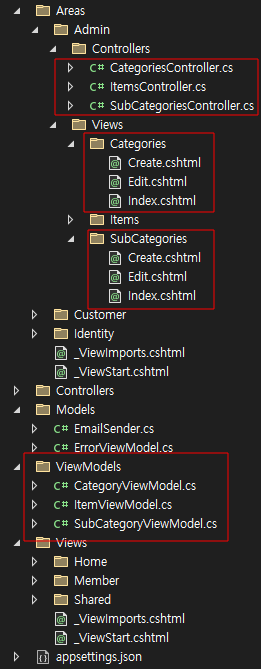
[ CategoryViewModel.cs 와 SubCategoryViewModel.cs ] - 입력폼 처리를 위한 ViewModel 클래스 생성
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
|
namespace FastFood.Web.ViewModels
{
public class CategoryViewModel
{
public int Id { get; set; }
public string Title { get; set; }
}
public class SubCategoryViewModel
{
public int Id { get; set; }
public string Title { get; set; }
public int CategoryId { get; set; } // FK
}
public class ItemViewModel
{
public int Id { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public double Price { get; set; }
public int CategoryId { get; set; }
public Category Category { get; set; }
public int SubCategoryId { get; set; }
public SubCategory SubCategory { get; set; }
public IFormFile ImageUrl { get; set; }
}
}
|
cs |
[ /Areas/Admin/Controllers/CategoriesController.cs ]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
|
using FastFood.Models;
using FastFood.Repository;
using FastFood.Web.ViewModels;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.Rendering;
namespace FastFood.Web.Areas.Admin.Controllers
{
[Area("Admin")]
public class CategoriesController : Controller
{
private readonly ApplicationDbContext _context;
public CategoriesController(ApplicationDbContext context)
{
_context = context;
}
[HttpGet]
public IActionResult Index()
{
var listFromDb = _context.Categories.ToList().Select(x=> new CategoryViewModel() {
Id = x.Id,
Title = x.Title
}).ToList();
return View(listFromDb);
}
[HttpGet]
public IActionResult Create()
{
CategoryViewModel category = new CategoryViewModel();
return View(category);
}
[HttpPost]
public IActionResult Create(CategoryViewModel vm)
{
Category model = new Category();
model.Title = vm.Title;
_context.Categories.Add(model);
_context.SaveChanges();
return RedirectToAction("Index");
}
[HttpGet]
public IActionResult Edit(int id)
{
var viewModel = _context.Categories.Where(x => x.Id == id)
.Select(x => new CategoryViewModel() { Id = x.Id, Title = x.Title }).FirstOrDefault();
return View(viewModel);
}
[HttpPost]
public IActionResult Edit(CategoryViewModel vm)
{
if (ModelState.IsValid)
{
var categoryFromDb = _context.Categories.FirstOrDefault(x=>x.Id==vm.Id);
if (categoryFromDb != null)
{
categoryFromDb.Title = vm.Title;
_context.Categories.Update(categoryFromDb);
_context.SaveChanges();
}
}
return RedirectToAction("Index");
}
[HttpGet]
public IActionResult Delete(int id)
{
var category = _context.Categories.Where(x => x.Id == id)
.FirstOrDefault();
if (category != null)
{
_context.Categories.Remove(category);
_context.SaveChanges();
}
return RedirectToAction("Index");
}
}
}
|
cs |
대분류 Category 의 CRUD Controller 부분 담았다. EF 방식으로 처리했다.
[ /Areas/Admin/Views/Categories/Create.cshtml ]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
|
@model FastFood.Web.ViewModels.CategoryViewModel
@{
ViewData["Title"] = "Create";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h1>Create</h1>
<h4>CategoryViewModel</h4>
<hr />
<div class="row">
<div class="col-md-4">
<form asp-action="Create">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<div class="form-group">
<label asp-for="Title" class="control-label"></label>
<input asp-for="Title" class="form-control" />
<span asp-validation-for="Title" class="text-danger"></span>
</div>
<div class="form-group">
<input type="submit" value="Create" class="btn btn-primary" />
</div>
</form>
</div>
</div>
<div>
<a asp-area="Admin" asp-controller="Categories" asp-action="Index">Back to List</a>
</div>
@section Scripts {
@{await Html.RenderPartialAsync("_ValidationScriptsPartial");}
}
|
cs |
[ /Areas/Admin/Views/Categories/Edit.cshtml ]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
|
@model FastFood.Web.ViewModels.CategoryViewModel
@{
ViewData["Title"] = "Edit";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h1>Edit</h1>
<h4>CategoryViewModel</h4>
<hr />
<div class="row">
<div class="col-md-4">
<form asp-action="Edit">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<div class="form-group">
<label asp-for="Id" class="control-label"></label>
<input asp-for="Id" class="form-control" />
<span asp-validation-for="Id" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="Title" class="control-label"></label>
<input asp-for="Title" class="form-control" />
<span asp-validation-for="Title" class="text-danger"></span>
</div>
<div class="form-group">
<input type="submit" value="Save" class="btn btn-primary" />
</div>
</form>
</div>
</div>
<div>
<a asp-action="Index">Back to List</a>
</div>
@section Scripts {
@{await Html.RenderPartialAsync("_ValidationScriptsPartial");}
}
|
cs |
[ /Areas/Admin/Views/Categories/Index.cshtml ]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
|
@model IEnumerable<FastFood.Web.ViewModels.CategoryViewModel>
@{
ViewData["Title"] = "Index";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h1>Category</h1>
<p>
<a asp-area="Admin" asp-controller="Categories" asp-action="Create">Create New</a>
</p>
<table class="table">
<thead>
<tr>
<th>
@Html.DisplayNameFor(model => model.Id)
</th>
<th>
@Html.DisplayNameFor(model => model.Title)
</th>
<th></th>
</tr>
</thead>
<tbody>
@if (Model != null)
{
foreach (var item in Model)
{
<tr>
<td>
@Html.DisplayFor(modelItem => item.Id)
</td>
<td>
@Html.DisplayFor(modelItem => item.Title)
</td>
<td>
@Html.ActionLink("Edit", "Edit", new { id=item.Id }) |
@Html.ActionLink("Details", "Details", new { id=item.Id }) |
@Html.ActionLink("Delete", "Delete", new { id=item.Id })
</td>
</tr>
}
}
</tbody>
</table>
|
cs |
지금까지 MVC 기법의 대분류 Category 부분을 CRUD 한 소스이다.
다음 "하" 편에서 본격적인 중분류 Select 박스 부분을 계속 정리하도록 하겠다.